In today's digital age, video communication has become an essential part of our daily lives. Whether it's for business meetings, online education, or social interactions, reliable and seamless video communication is crucial. This is where Dyte Video SDK comes into play, offering a powerful solution for integrating real-time video calling capabilities into your React Native application. In this blog, we'll walk you through the process of integrating the Dyte Video SDK with React Native, enabling you to build feature-rich video applications.
What is the Dyte Video SDK?
Dyte Video SDK is a developer-friendly tool that allows you to embed real-time video and audio communication features into your web and mobile applications. It offers a set of APIs and components that make it easy to create interactive and engaging video applications.
Prerequisites
Before we dive into the integration process, make sure you have the following prerequisites in place:
- React Native Environment: You should have a working React Native environment set up on your development machine. If you haven't already, follow the official React Native documentation to get started.
- Dyte Account: Sign up for a Dyte account on the Dyte website if you haven't already. You'll need your API credentials, which you can obtain from the Dyte dashboard.
- Node.js and npm: Ensure that you have Node.js and npm installed on your system. You can download them from the official Node.js website.
- React Native Dependencies: Initialise a React Native project with the required dependencies. You can do this using the
npx react-native init
command.
Steps to Integrate Dyte Video SDK with React Native
Now that you have the prerequisites in place let's go through the steps to integrate Dyte Video SDK into your React Native application.
Step 1: Create a New React Native Project
If you haven't already, create a new React Native project using the following command:
npx react-native init DyteVideoApp
Navigate to the project directory:
cd DyteVideoApp
Step 2: Install Dyte Video SDK and the dependencies
Install the Dyte Video SDK package using npm or yarn:
npm install @dytesdk/react-native-core
# or
yarn add @dytesdk/react-native-core
You can also install the UI-kit along with the core using:
npm install @dytesdk/react-native-ui-kit @dytesdk/react-native-core
Install the following dependencies:
npm install react-native-webrtc react-native-document-picker react-native-file-viewer react-native-fs react-native-safe-area-context react-native-sound-player react-native-svg react-native-webview
Step 3: Configure Dyte Credentials
You'll need to get you authtoken. Open your Dyte Developer portal and follow the steps here to get started with creating your Dyte authtokens.
Step 4: Initialize Dyte Video SDK
In your React Native code, initialize the Dyte Video SDK by importing it and initializing it with your Dyte credentials. Create a new JavaScript file (e.g., DyteVideo.js
) and add the following code:
import { useDyteClient } from '@dytesdk/react-native-core';
import { DyteUIProvider, DyteMeeting } from '@dytesdk/react-native-ui-kit';
export default function App() {
const [meeting, initMeeting] = useDyteClient();
useEffect(() => {
initMeeting({
authToken: '<auth-token>',
defaults: {
audio: false,
video: false,
},
});
}, []);
return (
<DyteProvider value={meeting}>
<DyteUIProvider>
<DyteMeeting meeting={meeting} />
</DyteUIProvider>
</DyteProvider>
);
}
Step 5: Use Dyte Video Components
Now, you can start using Dyte Video SDK components to create your video application. Add the necessary components and controls within the DyteVideoScreen
component you created in the previous step.
You can refer to the Dyte Video SDK documentation for a list of available components and their usage.
If you are using the UI kit directly, like us here, you can use Dyte’s prebuilt components. Here is an example of what’s going into this example:
function MyMeeting() {
const { meeting } = useDyteMeeting();
return (
<DyteProvider value={meeting}>
<DyteUIProvider>
<DyteMeeting meeting={meeting} />
</DyteUIProvider>
</DyteProvider>
);
}
Step 6: Run Your React Native Application
Start your React Native application to test the Dyte Video integration:
npx react-native run-android
# or
npx react-native run-ios
Make sure your Android or iOS simulator is running. Your application should then launch with the Dyte Video SDK integrated.
When to Use React Native
React Native is a great choice for mobile app development in various scenarios:
- Cross-Platform Development: When you need to target both iOS and Android platforms with a single codebase, React Native is a go-to framework. It allows you to write code once and run it on multiple platforms, saving development time and resources.
- Rapid Development: React Native's hot-reloading feature allows developers to see the results of their code changes almost instantly. This makes it ideal for fast-paced development environments and quick iterations.
- Access to Native Modules: React Native provides a bridge that enables you to access native modules when necessary. If your app requires functionality that is not available through React Native's core components, you can seamlessly integrate native code.
- Large and Active Community: React Native has a large and active community of developers. This means you can find a wealth of third-party libraries, components, and solutions to common problems, which can significantly speed up development.
- UI/UX Requirements: React Native is great for apps with complex and custom user interfaces. You can easily create native-like experiences with smooth animations and transitions.
When Not to Use React Native
While React Native is a powerful framework, it may not be the best choice for every mobile app project. Consider other options in the following situations:
- Highly Specialized Native Features: If your app relies heavily on platform-specific features or hardware-specific functionalities, such as advanced camera processing or ARKit/ARCore capabilities, it might be more practical to build natively using Swift (for iOS) or Java/Kotlin (for Android).
- Performance-Intensive Apps: Applications that require extremely high performance, such as complex 3D games, might not be suitable for React Native due to the overhead of the JavaScript bridge. In such cases, native development provides more control over performance optimization.
- Tight Deadlines: While React Native can accelerate development, if you have an extremely tight deadline and your team is already proficient in native development, sticking to native languages may be more efficient in the short term.
- Limited Third-Party Library Support: Although React Native has a rich ecosystem of libraries, there might be situations where you need access to niche or specialized native libraries that are not available through React Native.
- Strict Platform Guidelines: If your app must strictly adhere to platform-specific design guidelines (e.g., Material Design for Android and Human Interface Guidelines for iOS), you may encounter challenges ensuring pixel-perfect UI consistency with React Native.
Dyte's React Native SDK
Dyte's React Native SDK is a powerful tool that empowers developers to integrate real-time video and audio communication into their mobile applications. Here are some key features of Dyte's Video SDK:
- Cross-Platform Support: Dyte's React Native SDK supports both iOS and Android platforms, making it versatile for cross-platform app development.
- Customizable UI Components: Dyte provides customizable UI components, allowing you to create a video communication experience tailored to your app's design and branding.
- Scalability and Reliability: Dyte's infrastructure is built to handle scalability and offers high-quality video and audio streaming, ensuring a reliable communication experience for your users.
- Developer-Friendly APIs: The SDK offers developer-friendly APIs that make it easy to implement video communication features in your app. You can quickly set up video calls, chat, screen sharing, and more.
- Real-Time Collaboration: Dyte's SDK enables real-time collaboration features, making it suitable for a wide range of applications, including telemedicine, education, collaboration tools, and social networking.
- Security and Privacy: Dyte prioritizes security and privacy, offering end-to-end encryption for video and chat communication, ensuring that your users' data remains protected.
Conclusion
By integrating Dyte's React Native SDK into your mobile application, you can harness the power of real-time video communication and provide a seamless and engaging experience for your users, all while benefiting from the advantages of React Native's cross-platform development capabilities.
Further resources and reading
- Official Dyte SDK documentation: This is your go-to resource for any Dyte-related questions.
- Related articles or tutorials: Look for tutorials that offer more in-depth guidance on advanced Dyte SDK features.
- React best practices: Enhance your app's performance and user experience by following React's best practices.
Build Powerful Live Experiences 10x Faster
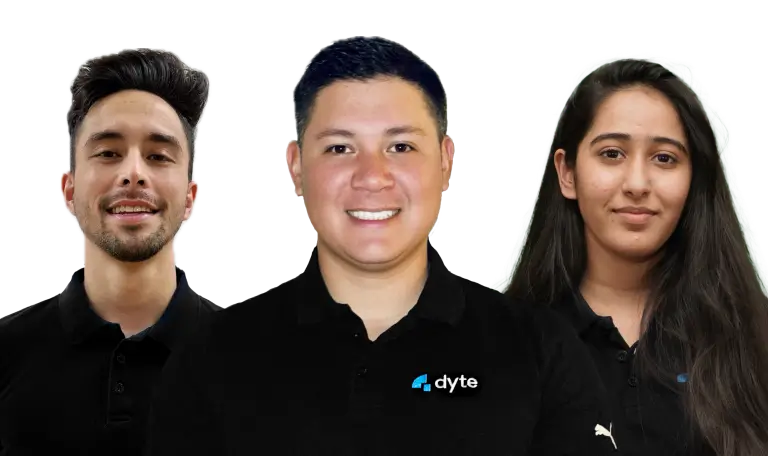