Our first mobile SDK was released around a year ago. While that SDK solved our problem but it came with certain limitations since it was made with React Native. Later when we needed to ship our native SDK, we wrapped React Native package inside the Android/iOS package and shipped the native SDK. When we needed a Flutter plugin we encapsulated Android/iOS libraries and our Flutter plugin was ready! After all of this wrapping, we had all the packages we needed but there were limitations to this approach.
The above solution got us our native Android SDK but it wasn’t up to the mark where we wanted it to be. Since this solution was not native on its core, every time a new client wanted to integrate this SDK into their native apps the size impact on their final binary was huge. This also resulted in a delay in load time as it need to boot up React Native engine.
Also, this SDK had a tight coupling of UI with business logic and hence we had minimum customizability for our clients. This was a better solution for clients who wanted complete tailored UI for video meetings, but not for those who needed a high amount of customizability.
Our learnings
After shipping the first SDK we got to learn that UI and business logic needs to be separated to achieve the customisability we didn’t have. This will also be easy for our clients as they will have a choice to either go with a full-blown solution or have just a core SDK and write their own UI.
Going ahead with React Native was the right choice back then but as we are scaling and gaining a lot of native clients, we also needed to go native to achieve high performance.
The size impact we had on the final binary is a big factor we consider. And with the older approach, native mobile apps were not treated fairly, We need to do something better here!
Time for a new SDK!
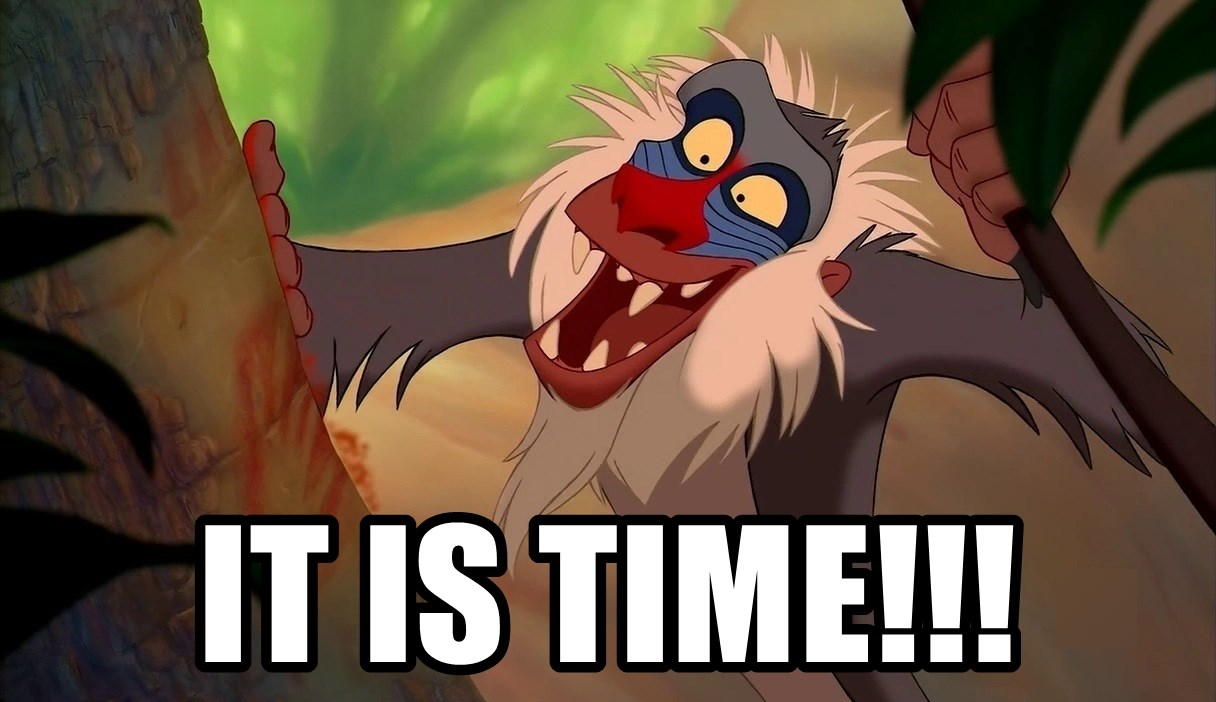
This time we wanted to do something better and more importantly go native! Hence we decided to go ahead with Kotlin Multiplatform. This allows us to write business logic once and use it on both Android and iOS. Support for multiplatform programming is one of Kotlin’s key benefits. It reduces time spent writing and maintaining the same code for different platforms while retaining the flexibility and benefits of native programming. Sharing code between mobile platforms is one of the major Kotlin Multiplatform use cases. With Kotlin Multiplatform Mobile(KMM), you can build cross-platform mobile applications and share common code between Android and iOS, such as business logic, connectivity, and more.
With the help of this approach, we were able to keep our networking/state management code in common and share it between Android/iOS and yet it allows us to write native code wherever required. This approach is way better than writing two different SDKs as we can expect the same behavior in both the targets. Since we are building an SDK, using Kotlin Multiplatform forces us to use keep exact same contracts for both Android and iOS which results in much more consistent output without putting much more effort into it.
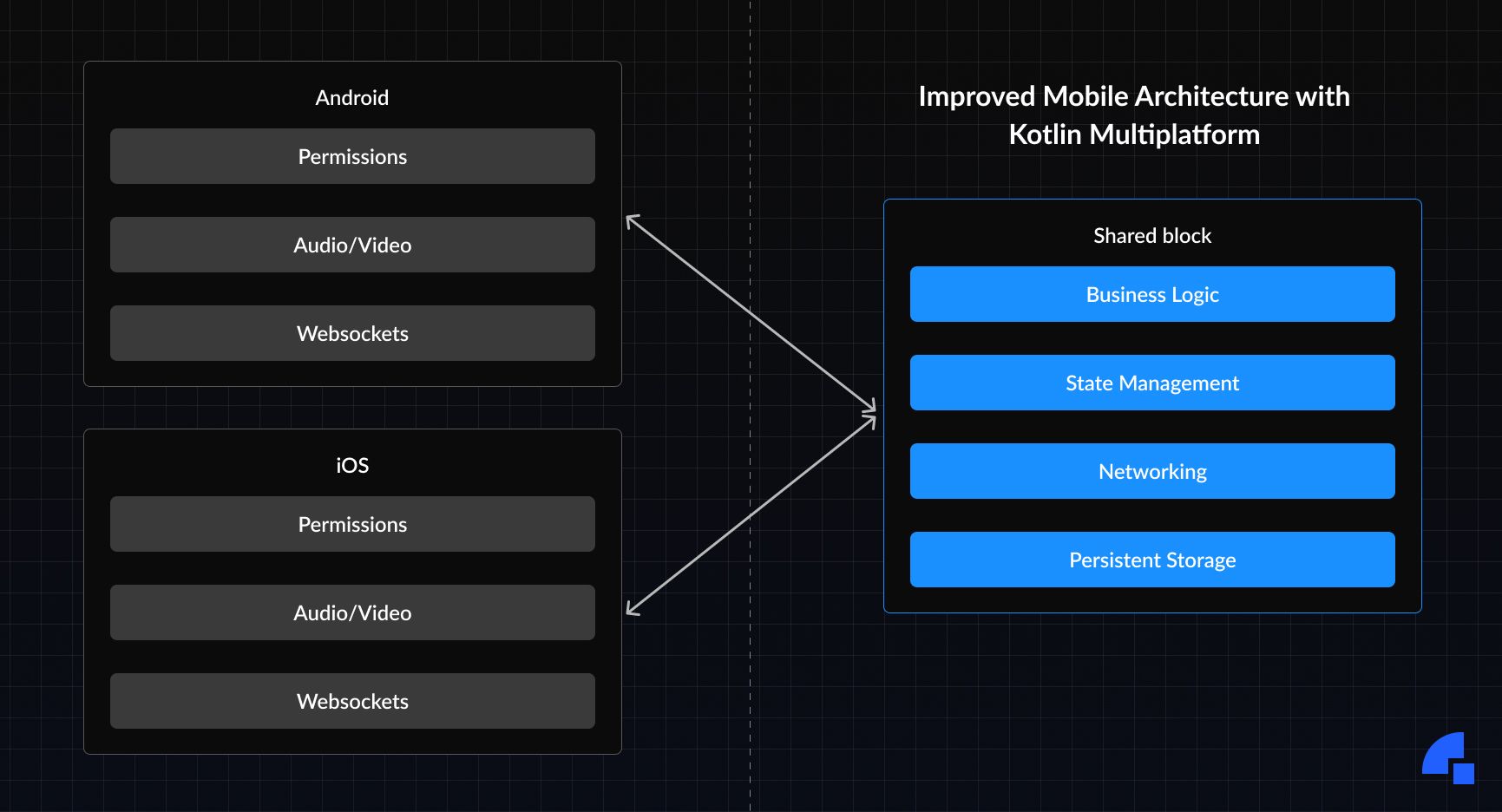
This time we decided to break the new mobile SDK into two main components. Mobile Core and Mobile UI kit (more on this later, stay tuned!).
The mobile core will cater to the data-only layer and allows you to build your own UI from scratch. While at the same time still easy to use, providing high-level primitives and abstracting all complex media and networking optimizations from the end-user. This SDK will have simple APIs for you to be able to join in a meeting with minimum code and maximum configuration. Since this SDK is made natively it’ll only add required classes to your final binary resulting in minimum size impact. The load time will be much faster as UI is rendered separately from the core.
Anatomy of the SDK
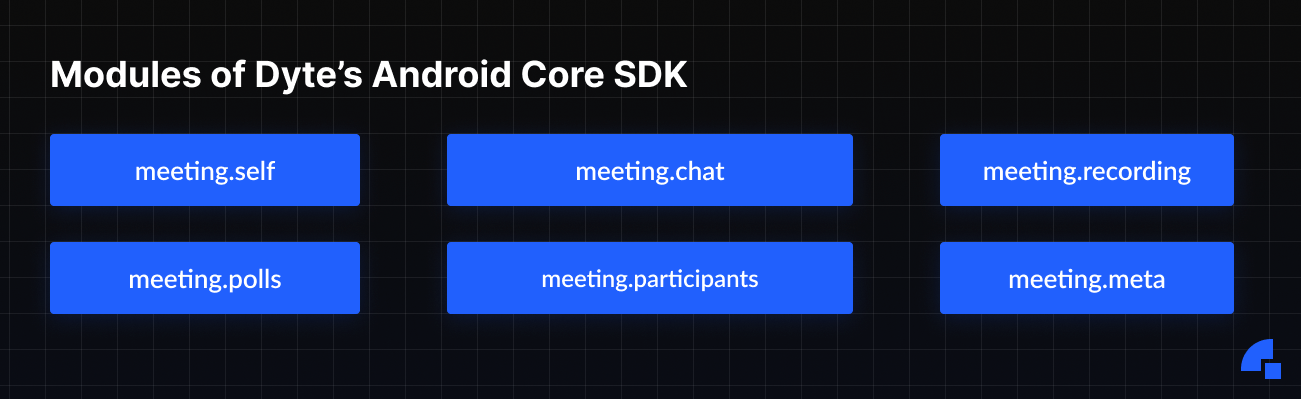
We’ve tried to make composite high-level modules such as the ones mentioned above. Here are all the modules that our SDK consists of:
- meeting:
DyteMobileClient
is the main entry point for your app to be able to talk to Dyte SDK. To get an instance ofDyteMobileClient
you can make use of the builder provided in the package itself. - meeting.self: This consists of properties and methods corresponding to the current (local) user, such as enabling or disabling their audio and video, or changing their device.
- meeting.participants: This module is useful for providing information about the other participants that are present in the meeting. A host can use this module for access control, i.e., the host can mute or kick a participant.
- meeting.chat: Consists of actions that you can perform on the meeting chat, such as sending, receiving, and deleting messages.
- meeting.polls: This module lets you perform actions related to polls.
- meeting.recording: This lets you start or stop a recording, and get the current status of an ongoing recording.
- Every operation you do on
DyteMobileClient
will trigger an appropriate event for you. There are four types of event listeners you can subscribe to i.e self, meeting, participants, hardware(camera/microphone) events.
How to use Android Core SDK
- To install the SDK use
implementation 'io.dyte.core:shared:+'
- To get an instance of
DyteMobileClient
you can make use of the builder provided in the package itself.
val meeting = DyteAndroidClientBuilder.build(activity)
- To be able to join in a meeting you need to construct an object of
DyteMeetingInfo
like following
val meetingInfo = DyteMeetingInfo(
orgId = ORGNIZATION_ID,
roomName = MEETING_ROOM_NAME,
authToken = AUTH_TOKEN,
)
- Once you have access to
DyteMeetingInfo
you need to callinit()
method on themeeting
object.
meeting.init(meetingInfo)
- Now one last step is to call
joinRoom()
meeting.joinRoom()
- Once you’re in the meeting room name, doing the rest of the operations is a piece of cake. Now if you want to mute yourself in a meeting, all you need to is
meeting.self.disableAudio()
- Similarly, if you want to send a chat message all you need is
meeting.chat.sendTextMessage(”hello, this is the new way!”)
.
We have built a sample app using this same SDK and you can find the source code here. You can read more about this SDK in our documentation.
Do reach out to us if you have any feedback or if you need any help integrating Dyte into your mobile applications.
If you haven’t heard about Dyte yet, head over to https://dyte.io to learn how we are revolutionizing live video calling through our SDKs and libraries and how you can get started quickly on your 10,000 free minutes which renew every month. If you have any questions, you can reach us at support@dyte.io or ask our developer community.