In today’s digital landscape, the art of buying and selling has taken a remarkable leap forward with the advent of real-time online marketplaces and auctions. A live auction is an interactive bidding process in real-time, facilitated through digital platforms. Participants place live bids on goods or services within a defined timeframe, and the highest bid at the end of the auction wins the item or service being auctioned.
We want to help people create engaging live experiences with Dyte, and live auctions just happened to be on the market. By blending technology with real-time bidding, in this blog, we’ll delve into the essentials and take you through the application flow of building and running your own live auction platform before the fall of the hammer.
Let’s discover the products up for sale before the bidding starts!
Why build a live auction platform?
- Convenient: Participate in live auctions from anywhere, at any time.
- Real-time bidding: Experience the thrill of bidding against other enthusiasts in real-time.
- Wide range of items: Discover diverse items, from collectibles to artwork and more.
- Seamless integration: The live auction app provides users with a smooth and uninterrupted auction experience with Dyte's reliable audio/video conferencing and customizable UI.
Before you start
- Basic knowledge of React.js is required to build this application.
- Please ensure that Node.js is installed on your machine. We will use it to run our application.
- Lastly, you will need the API Key and organization ID from the developer portal to authenticate yourself when you call Dyte's REST APIs.
Building the live bidding platform
Installation
You need to install Dyte's React UI Kit and Core packages to get started. You can do so by using npm or Yarn.
npm install @dytesdk/react-ui-kit @dytesdk/react-web-core
Getting started
We will first fetch the organization ID and API Key from the developer portal. Then, we'll create an account and navigate to the API Keys page.
Please ensure that you do not upload your API Key anywhere.
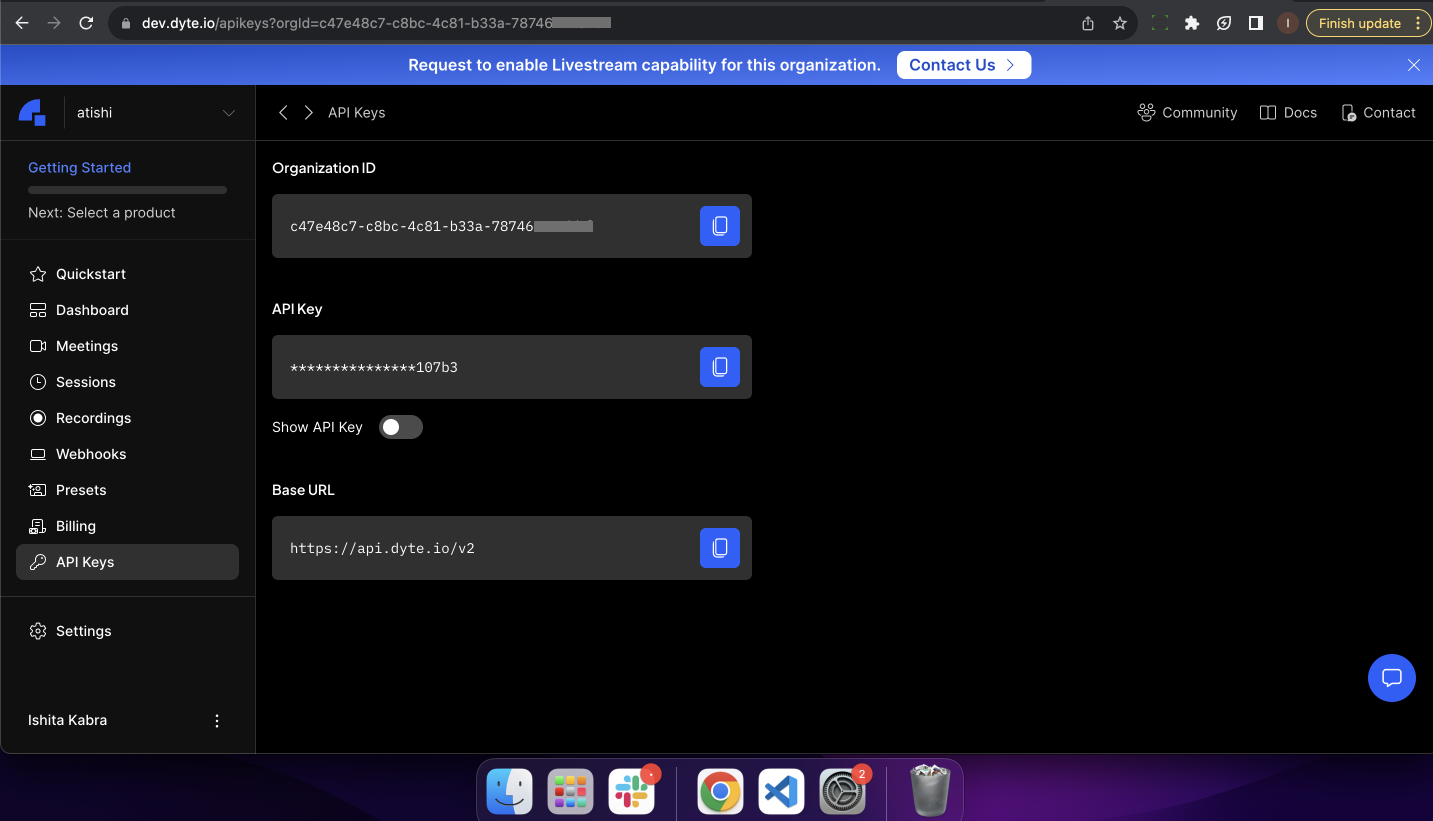
Next, we will create a meeting using the following Rest API. Here is a sample response.
{
"success": true,
"data": {
"id": "497f6eca-6276-4993-bfeb-53cbbbbaxxxx",
"name": "string",
"picture": "<http://example.com>",
"custom_participant_id": "string",
"preset_name": "string",
"created_at": "2019-08-24T14:15:22Z",
"updated_at": "2019-08-24T14:15:22Z",
"token": "string"
}
}
We use the ID from the payload to generate an auth token using the Add Participants API. This auth token is used to initialize a Dyte client. Let's start setting up the project.
Build your custom UI
Create a file src/App.tsx
. We will use the DyteMeeting
hook to initialize a new Dyte meeting. The DyteProvider
is used to pass the meeting object to all child components inside this application. We will also set up event listeners for joining and leaving the room.
import { useEffect, useState } from 'react';
import { DyteProvider, useDyteClient } from '@dytesdk/react-web-core';
import { LoadingScreen } from './pages';
import { Meeting, SetupScreen } from './pages';
function App() {
const [meeting, initMeeting] = useDyteClient();
const [roomJoined, setRoomJoined] = useState<boolean>(false);
useEffect(() => {
const searchParams = new URL(window.location.href).searchParams;
const authToken = searchParams.get('authToken');
if (!authToken) {
alert(
"An authToken wasn't passed, please pass an authToken in the URL query to join a meeting."
);
return;
}
initMeeting({
authToken,
defaults: {
audio: false,
video: false,
},
});
}, []);
useEffect(() => {
if (!meeting) return;
const roomJoinedListener = () => {
setRoomJoined(true);
};
const roomLeftListener = () => {
setRoomJoined(false);
};
meeting.self.on('roomJoined', roomJoinedListener);
meeting.self.on('roomLeft', roomLeftListener);
return () => {
meeting.self.removeListener('roomJoined', roomJoinedListener);
meeting.self.removeListener('roomLeft', roomLeftListener);
}
}, [meeting])
return (
<DyteProvider value={meeting} fallback={<LoadingScreen />}>
{
!roomJoined ? <SetupScreen /> : <Meeting />
}
</DyteProvider>
)
}
Now, we will build a setup screen; this is the first page that the user will see.
Create a file src/pages/setupScreen/setupScreen.tsx
. We will update the user's display name and join the Dyte meeting from this page.
import { useEffect, useState } from 'react'
import './setupScreen.css'
import { useDyteMeeting } from '@dytesdk/react-web-core';
import {
DyteAudioVisualizer,
DyteAvatar,
DyteCameraToggle,
DyteMicToggle,
DyteNameTag,
DyteParticipantTile,
} from '@dytesdk/react-ui-kit';
const SetupScreen = () => {
const { meeting } = useDyteMeeting();
const [isHost, setIsHost] = useState<boolean>(false);
const [name, setName] = useState<string>('');
useEffect(() => {
if (!meeting) return;
const preset = meeting.self.presetName;
const name = meeting.self.name;
setName(name);
if (preset.includes('host')) {
setIsHost(true);
}
}, [meeting])
const joinMeeting = () => {
meeting?.self.setName(name);
meeting.joinRoom();
}
return (
<div className='setup-screen'>
<div className="setup-media">
<div className="video-container">
<DyteParticipantTile meeting={meeting} participant={meeting.self}>
<DyteAvatar size="md" participant={meeting.self}/>
<DyteNameTag meeting={meeting} participant={meeting.self}>
<DyteAudioVisualizer size='sm' slot="start" participant={meeting.self} />
</DyteNameTag>
<div className='setup-media-controls'>
<DyteMicToggle size="sm" meeting={meeting}/>
 
<DyteCameraToggle size="sm" meeting={meeting}/>
</div>
</DyteParticipantTile>
</div>
</div>
<div className="setup-information">
<div className="setup-content">
<h2>Welcome! {name}</h2>
<p>{isHost ? 'You are joining as a Host' : 'You are joining as a bidder'}</p>
<input disabled={!meeting.self.permissions.canEditDisplayName ?? false} className='setup-name' value={name} onChange={(e) => {
setName(e.target.value)
}} />
<button className='setup-join' onClick={joinMeeting}>
Join Meeting
</button>
</div>
</div>
</div>
)
}
export default SetupScreen
Now that we have the basic setup let's build the live auction platform.
Create a file src/pages/meeting/Meeting.tsx
.
Our live auction app will implement the following functionality:
- Give hosts an option to start/stop the auction.
- Give hosts an option to navigate between different auction products.
- Allow users to make real-time bids for each product.
- Show the highest bid to all users.
import { useEffect, useState } from 'react'
import './meeting.css'
import {
DyteCameraToggle,
DyteChatToggle,
DyteGrid,
DyteHeader,
DyteLeaveButton,
DyteMicToggle,
DyteNotifications,
DyteParticipantsAudio,
DyteSidebar,
sendNotification,
} from '@dytesdk/react-ui-kit'
import { useDyteMeeting } from '@dytesdk/react-web-core';
import { AuctionControlBar, Icon } from '../../components';
import { bidItems } from '../../constants';
interface Bid {
bid: number;
user: string;
}
const Meeting = () => {
const { meeting } = useDyteMeeting();
const [item, setItem] = useState(0);
const [isHost, setIsHost] = useState<boolean>(false);
const [showPopup, setShowPopup] = useState<boolean>(true);
const [auctionStarted, setAuctionStarted] = useState<boolean>(false);
const [activeSidebar, setActiveSidebar] = useState<boolean>(false);
const [highestBid, setHighestBid] = useState<Bid>({ bid: 100, user: 'default' });
const handlePrev = () => {
if (item - 1 < 0) return;
setItem(item - 1)
meeting.participants.broadcastMessage('item-changed', { item: item - 1 })
}
const handleNext = () => {
if ( item + 1 >= bidItems.length) return;
setItem(item + 1)
meeting.participants.broadcastMessage('item-changed', { item: item + 1 })
}
useEffect(() => {
setHighestBid({
bid: bidItems[item].startingBid,
user: 'default'
})
}, [item])
useEffect(() => {
if (!meeting) return;
const preset = meeting.self.presetName;
if (preset.includes('host')) {
setIsHost(true);
}
const handleBroadcastedMessage = ({ type, payload }: { type: string, payload: any }) => {
switch(type) {
case 'auction-toggle': {
setAuctionStarted(payload.started);
break;
}
case 'item-changed': {
setItem(payload.item);
break;
}
case 'new-bid': {
sendNotification({
id: 'new-bid',
message: `${payload.user} just made a bid of $ ${payload.bid}!`,
duration: 2000,
})
if (parseFloat(payload.bid) > highestBid.bid) setHighestBid(payload)
break;
}
default:
break;
}
}
meeting.participants.on('broadcastedMessage', handleBroadcastedMessage);
const handleDyteStateUpdate = ({detail}: any) => {
if (detail.activeSidebar) {
setActiveSidebar(true);
} else {
setActiveSidebar(false);
}
}
document.body.addEventListener('dyteStateUpdate', handleDyteStateUpdate);
return () => {
document.body.removeEventListener('dyteStateUpdate', handleDyteStateUpdate);
meeting.participants.removeListener('broadcastedMessage', handleBroadcastedMessage);
}
}, [meeting])
useEffect(() => {
const participantJoinedListener = () => {
if (!auctionStarted) return;
setTimeout(() => {
meeting.participants.broadcastMessage('auction-toggle', {
started: auctionStarted
})
}, 500)
}
meeting.participants.joined.on('participantJoined', participantJoinedListener);
return () => {
meeting.participants.joined.removeListener('participantJoined', participantJoinedListener);
}
}, [meeting, auctionStarted])
const toggleAuction = () => {
if (!isHost) return;
meeting.participants.broadcastMessage('auction-toggle', {
started: !auctionStarted
})
if (!auctionStarted) {
meeting.self.pin();
} else {
meeting.self.unpin();
}
setAuctionStarted(!auctionStarted);
}
return (
<div className='meeting-container'>
<DyteParticipantsAudio meeting={meeting} />
<DyteNotifications meeting={meeting} />
<DyteHeader meeting={meeting} size='lg'>
<div className="meeting-header">
{
auctionStarted && (
<div className="show-auction-popup" onClick={() => setShowPopup(() => !showPopup)}>
<Icon size='sm' icon={showPopup ? 'close' : 'next'} />
</div>
)
}
</div>
</DyteHeader>
<div className='meeting-grid'>
{
auctionStarted && (
<div className={`auction-container ${!showPopup ? 'hide-auction-popup' : ''}`}>
<img className='auction-img' src={bidItems[item].link} />
<div className='auction-desc'>
{bidItems[item].description}
</div>
<AuctionControlBar
item={item}
highestBid={highestBid}
handleNext={handleNext}
handlePrev={handlePrev}
isHost={isHost}
/>
</div>
)
}
<DyteGrid layout='column' meeting={meeting} style={{ height: '100%' }}/>
{activeSidebar && <DyteSidebar meeting={meeting} />}
</div>
<div className='meeting-controlbar'>
<DyteMicToggle size='md' meeting={meeting} />
<DyteCameraToggle size='md' meeting={meeting} />
<DyteLeaveButton size='md' />
<DyteChatToggle size='md' meeting={meeting} />
{
isHost && (
<button className='auction-toggle-button' onClick={toggleAuction}>
<Icon size='lg' icon='auction' />
{auctionStarted ? 'Stop' : 'Start'} Auction
</button>
)
}
</div>
</div>
)
}
export default Meeting
Et voila! Our live auction app is ready.
You can play around with this live bidding app to get you going with some real-time bidding at - https://dyte-live-bidding.vercel.app/! Dyte going once, going twice...
You can check out the complete code for the project here.
Are you sold yet, or do we need to say more?
Conclusion
With this, we have built our live auction platform. All you have to do is pull out your gavel and shout at the top of your lungs. We look forward to you outbidding us!
If you have any thoughts or feedback, please reach out to us on LinkedIn and Twitter. Stay tuned for more related blog posts in the future!
Get better insights on leveraging Dyte's technology and discover how it can revolutionize your app's communication capabilities with its SDKs. Head over to dyte.io to learn how to start quickly on your 10,000 free minutes, which renew every month. You can reach us at support@dyte.io or ask our developer community if you have any questions.