React has its own unique design patterns, there is a certain style to how developers build on React, from the lifecycle of components to the functional programming paradigms of hooks.
So when it comes to adding live video/audio experiences within an App, a developer should not need to move away from these abstractions. Developing video calling applications shouldn’t be full of glue code mapping a library made with other design patterns to React’s patterns.
That is why we are launching two SDKs targeted specifically for React.
- React Web Core
- React UI Kit
Flux-like Design Pattern
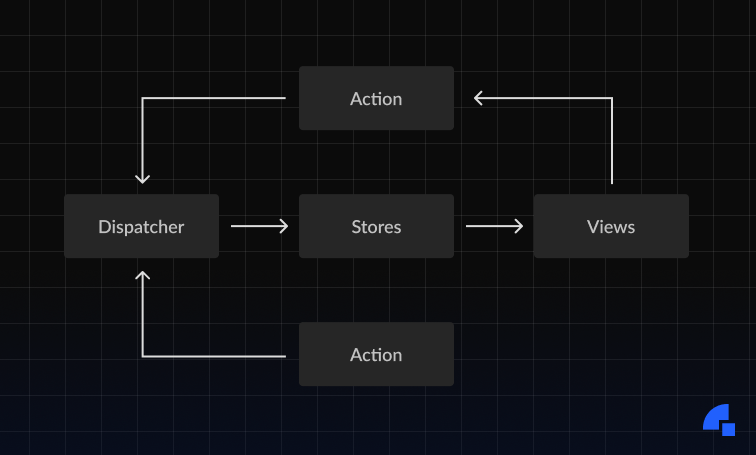
Flux is an architectural pattern proposed by Facebook for building React apps. The pattern describes a unidirectional data flow and has the following components
- Store
- Dispatcher/Actions
- Views
To implement this pattern our SDK exposes two hooks
1. useDyteMeeting
const { meeting } = useDyteMeeting();
All the actions that you need to take in a meeting, would be executed on this object
// To mute
meeting.self.disableAudio();
// To unmute
meeting.self.enableAudio();
2. useDyteSelector
All the meeting state is managed by one central store, to consume the data we provide the following hook
const audioEnabled = useDyteSelector((meeting) => meeting.self.audioEnabled);
If you have ever used redux, this should feel right at home. useDyteSelector
will only cause a re-render when the data is updated. For example
meeting.self.enableAudio();
// the below hook will not cause the component to re-render
const videoEnabled = useDyteSelector((meeting) => meeting.self.videoEnabled);
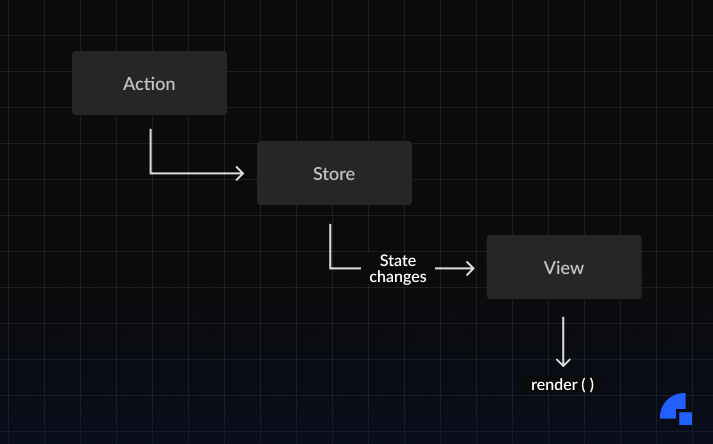
You can also take action on the changes in these data
const { meeting } = useDyteMeeting();
const roomJoined = useDyteSelector((m) => m.self.roomJoined);
useEffect(() => {
if(roomJoined) {
meeting.chat.sendTextMessage(`Hello`);
}
},[roomJoined]);
You can also listen to events the regular way using event listeners
useEffect(() => {
const onJoin = (p) => {
console.log(`${p.name} joined`);
});
meeting.participants.joined.on('participantJoin', onJoin);
return () => meeting.participants.joined.removeListener(onJoin);
},[]);
For these hooks to work they need a parent Context Provider
function App() {
const [ client, initClient ] = useDyteClient();
useEffect(() => {
initClient({
roomName,
authToken
});
}, []);
return (
<DyteProvider value={client}>
<YourMeetingComponent />
</DyteProvider>
);
}
For more information read our docs https://docs.dyte.io/react-ui-kit/basics/using-hooks.
Now how do you build User Interfaces with this SDK?
Effortlessly
React UI Kit
In case you missed our last blog on the UI Kit, read it here https://dyte.io/blog/custom-ui-kit-sdk/
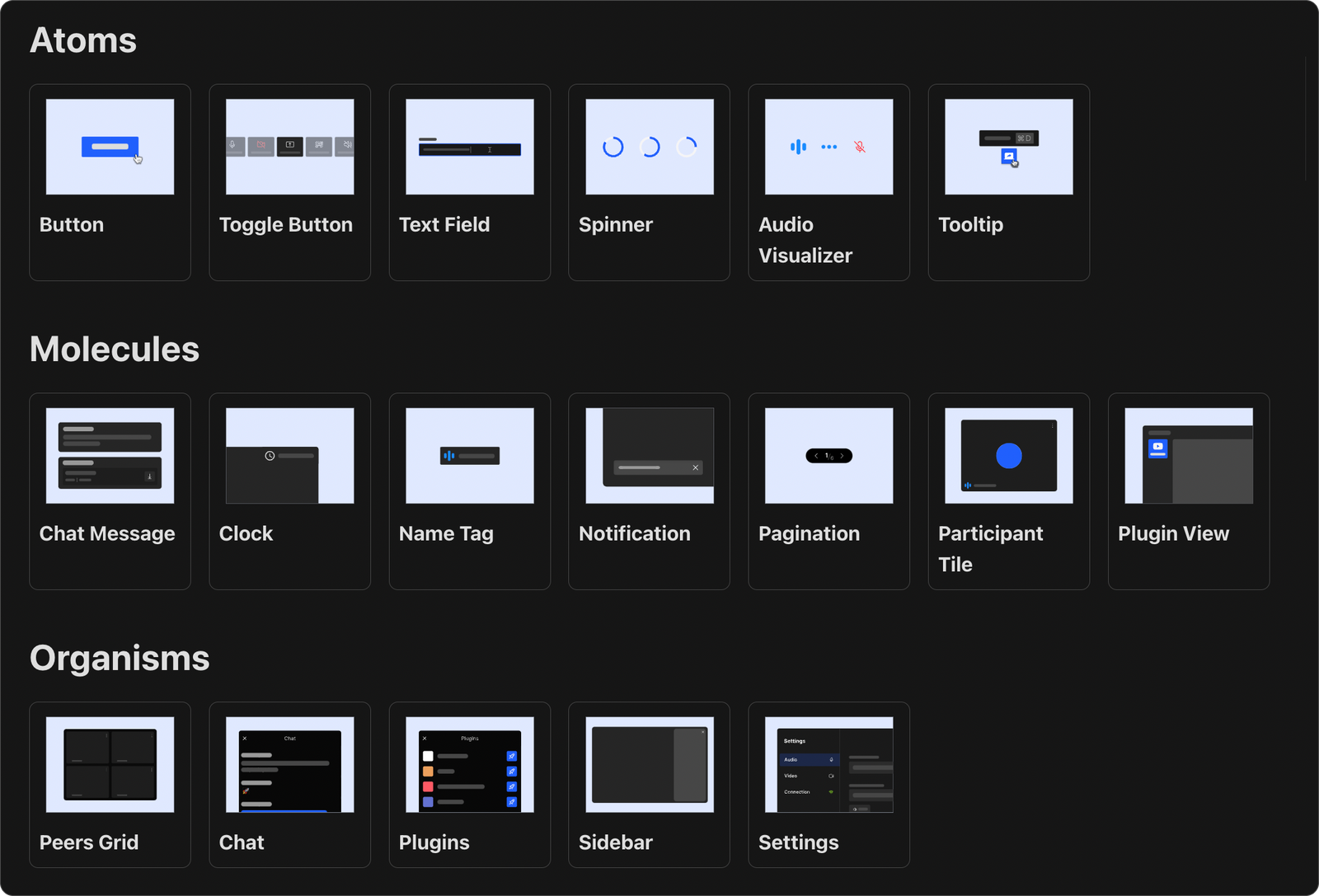
Our React UI Kit works seamlessly with the core objects. While our default UI is one line of code
<DyteMeeting meeting={meeting} />
With just a few more lines of code, you can customise it however you want, for example, if you want your participant tile to have a google meet style pin button on hover, you can make your own tile in 10 lines of code.
function ParticipantTile({ children, participant, size, ...rest }: any) {
return (
<DyteParticipantTile participant={participant} size={size} {...rest}>
<DyteAvatar participant={participant} size={size} />
<DyteNameTag participant={participant} size={size}>
<DyteAudioVisualizer participant={participant} size={size} />
</DyteNameTag>
<button className="pin-btn" onClick={() => participant.pin()}>
<PinIcon />
</button>
</DyteParticipantTile>
);
}
And use it with objects from useDyteSelector
const self = useDyteSelector((meeting) => meeting.self);
return (<ParticipantTile participant={self} id="self-tile" size="sm" />);
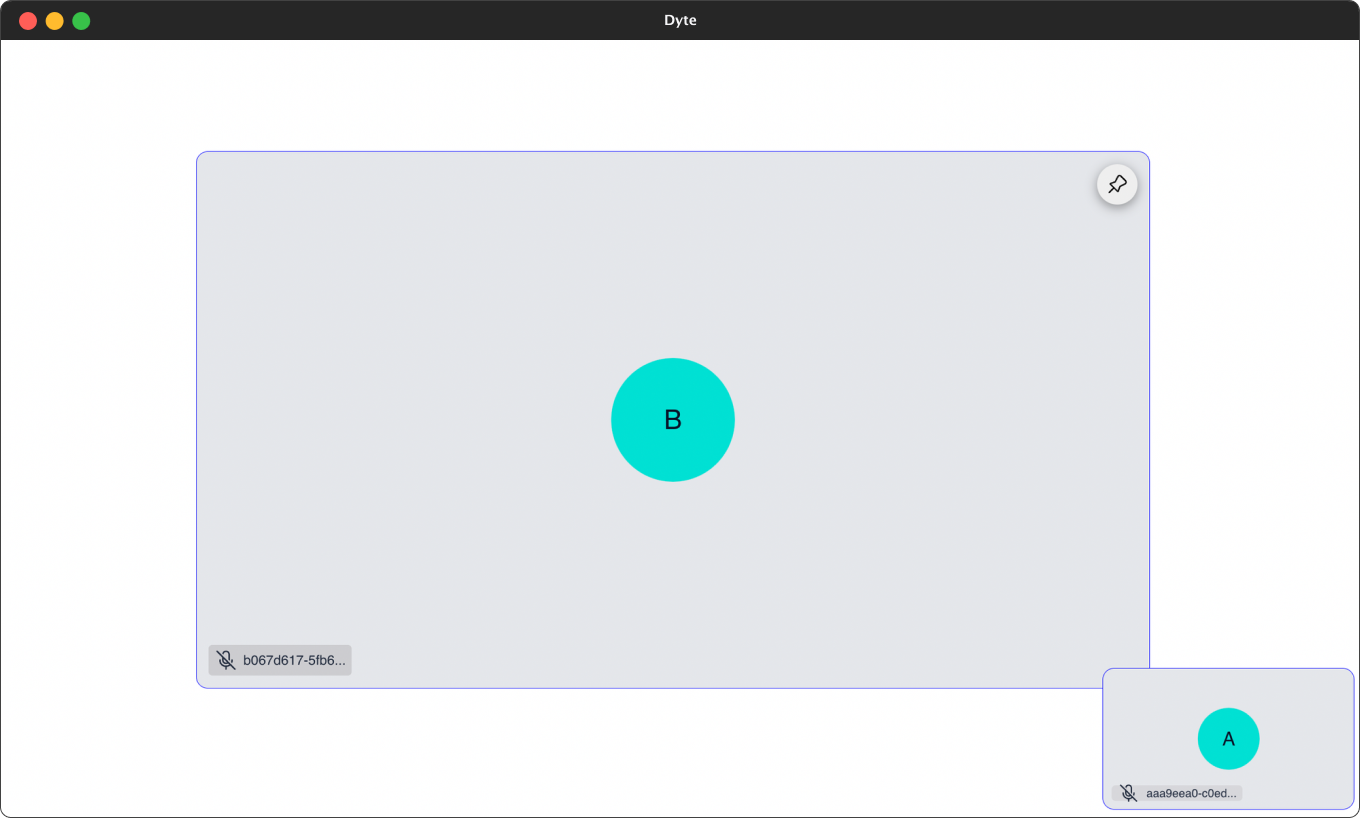
Powerful, Customizable and Easy to Use
Read more about our React Core SDK and React UI Kit at docs.dyte.io
If you haven’t heard about Dyte yet, head over to https://dyte.io to learn how we are revolutionizing live video calling through our SDKs and libraries and how you can get started quickly on your 10,000 free minutes which renew every month. If you have any questions, you can reach us at support@dyte.io or ask our developer community.